Introduction
Loading dynamic web page is very easy using JQuery and Ajax.In this article we will detailled the mechanisme adopted to load dynamic HTML data in web Page with Asynchronous mode thanks to Ajax.
Background
This article may be useful for intermediate developers who have some basics in HTML, Ajax, JQuery, JavaScript, Entity Framework, C# and ASP.NET MVC.I) Operation Process
In this section we will good explain the mechanism adopted to implement a such process.Before we start speak about the process, i want to do a reminder about MVC pattern and Ajax concept.
a) Reminder :
1. MVC :
(Model View Controller) is a pattern model most useful for developping web application.
The pattern model is tried and porven solution to separate information display, user actions and data access. where it improves the testability and code maintenance.
We can well understand the principle of this pattern through the next diagram.
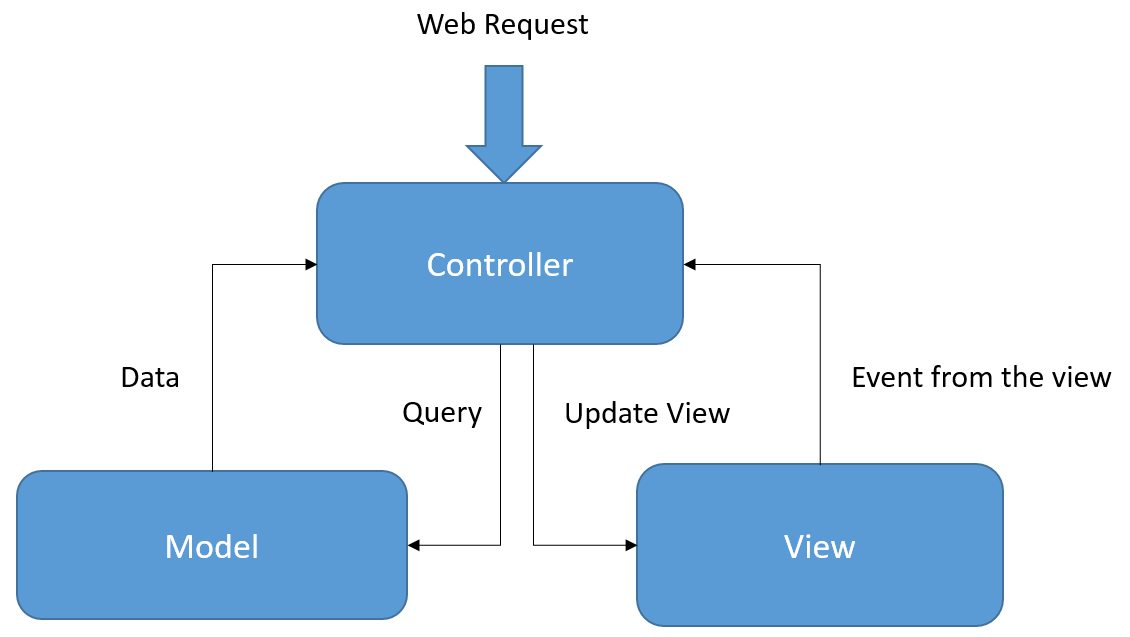
- Model : dispose of the source data.
- View : represent a user interface.
- Controller : handles a request from a view, updates the model and view state.
AJAX (Asynchronous JavaScript And XML) is concept of programming that combine different technologies such as the javaScript and XML.
The main idea of AJAX, is to ensure the communication between server and web page without causing a page reload.
To better understand the AJAX concept, we will show you a diagram that present the difference between classic web application and AJAX web application.

- reduce latency,
- avoid reloading web page,
- increase the reactivity of web page.
b) Dynamic load diagram
In this section we will detail the different interaction and tasks acheived between Client and Server to give a dynamic rendering.
the dynamic load can goes through the following basic steps :
1) constuction of data (html + css) whatever from client or server side,
2) insert the data into html tag with the help of javaScript.
Let see an example of code :
to use this example you must import the Jquery library
<script src="https://ajax.googleapis.com/ajax/libs/jquery/1.11.3/jquery.min.js"></script>
- JavaScript Code
<script>
$(document).ready(function(){
//detect the clic event on the button
$("button").click(function(){
//intialize html data as string
var htmlData = "NASRI";
//insert data into span block
$("#span1").html(htmlData );
});
});
</script>
- Html code to insert into Body tag
My name is </br><button>clic to display name</button>
the result will be :

the next diagram, explain better the mechanism of dynamic display, where the HTML data are composed in server side and returned to client for only display through javaScript instructions.
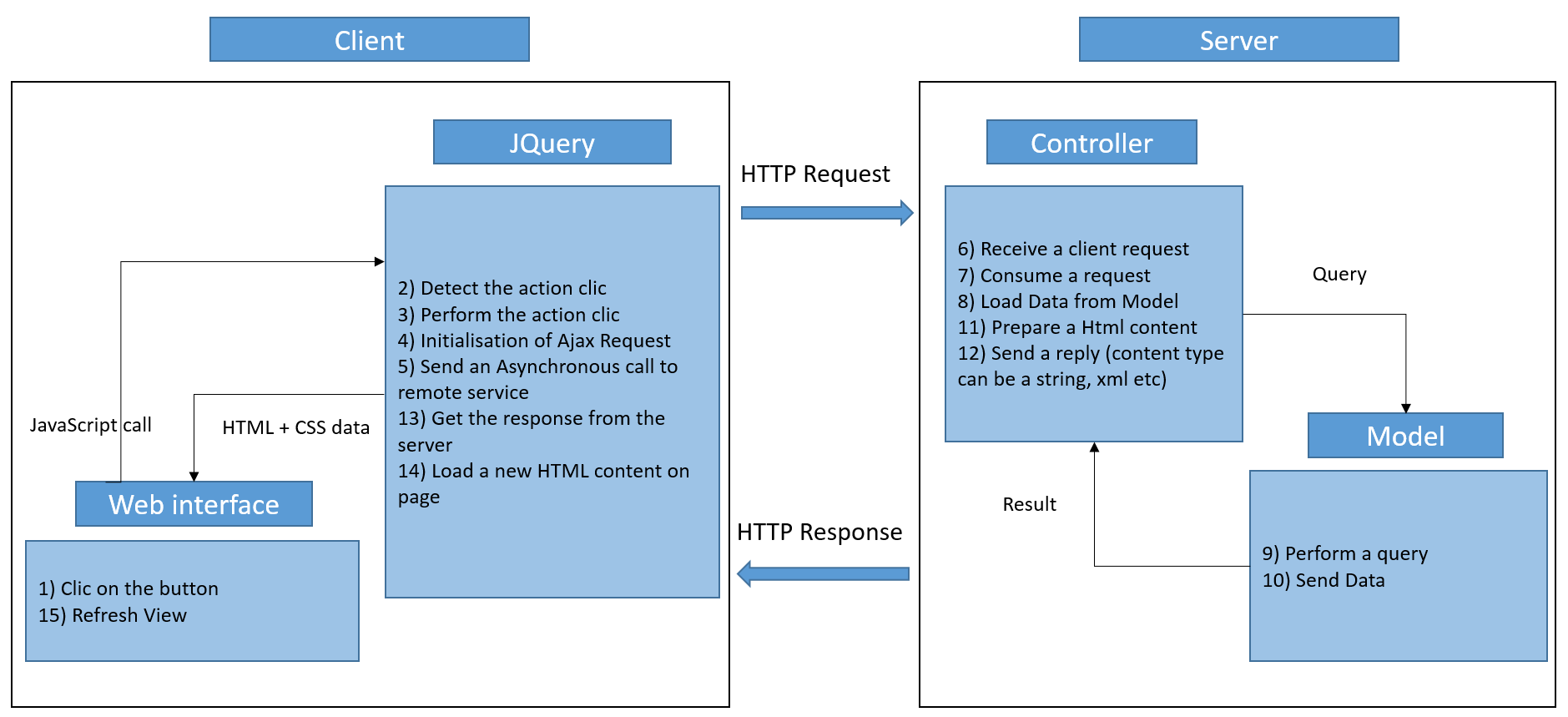
II) Example of Implementation
In this level, we will explain how implement the dynamic load through an ASP.NET MVC example.
a) About :
The adopted example, allow a user when he clic on a button, to load dynamic data (HTML + CSS) from a local DataBase.
The HTML block is composed by : 'static image', 'Product Label' and 'Product description' loaded from the Product Table.
In this example we used a local DataBase (.mdf)
b) Steps :
1) Creation of Local DataBase (.mdf)
We will show the differents steps to create the local dataBase (.mdf) through printed screen :





After creation of dataBase , we will use an ORM (object-relational mapping (Entity = Table) ) framework for ADO.NET named Entity Framework (EF) .
EF gives a possibility to query a database through instance of entities without write any sql statement.
In our example we will use EF Database First approch (we start from an existing DataBase to have entities (object)) :



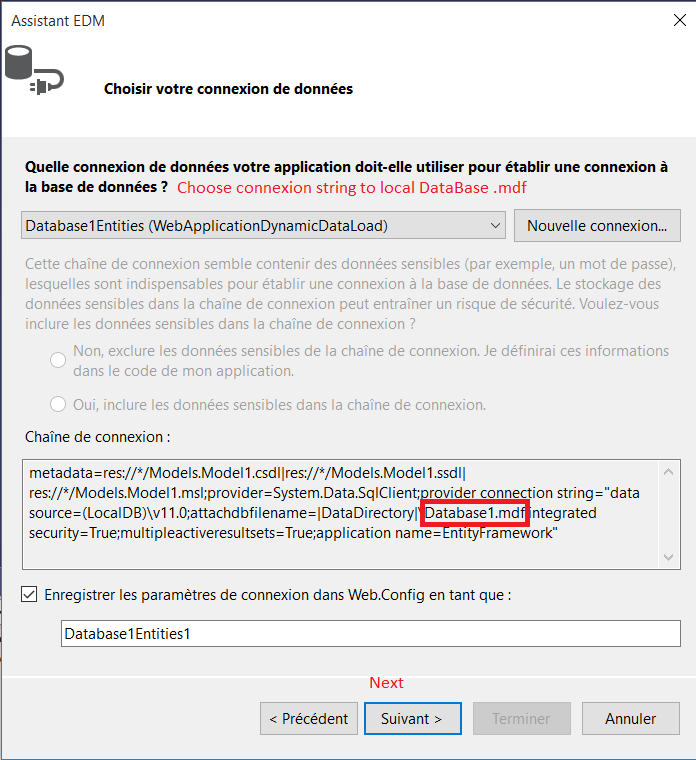


3) Implementation
In this section, we start the coding part, at the begining we comment the javascript code, then html and c# Asp.net MVC code.
- JavaScript Code :
function initContent() {
//Clear Screen by putting the following message
$("#idDivProducts").html("Empty Content, clic on Scan Button to load products");
}
$(document).ready(function () {
initContent();
});
//JQuery code that detect the click on Scan Button
$(document).on("click", "#idBtResetProducts", function () {
initContent();
});
//JQuery code that detect the click on Scan Button
$(document).on("click", "#idBtScanProducts", function () {
//url of service
var url = "/Home/GetProducts";
$.ajax({
type: "POST",//choose your HTTP request type ('POST' or 'GET')
url: url,
success: function (data) {
//display data (HTML+CSS) into html block
$("#idDivProducts").html(data);
},
error: function (xhr, ajaxOptions, thrownError) {
//display error message into html block
$("#idDivProducts").html("Echec Loading");
}
});
});
- HTML Code :
<div class="col-lg-12">
<button class="btn btn-default" id="idBtScanProducts">Scan Products</button>
<button class="btn btn-default" id="idBtResetProducts">Rest Products</button>
</div>
<hr>
<div id="idDivProducts" class="row">
<!--Our container : will get the output result (html data)-->
</div>
- C# Asp.net MVC Code :
public string GetProducts()
{
StringBuilder dataHTML = new StringBuilder();
//Entity framework
//Create Data Context of Data Base
WebApplicationDynamicDataLoad.Models.Database1Entities1 dataBaseEntities =
new Models.Database1Entities1();
//Get All Product
var listProduct = dataBaseEntities.Product.ToList();
//Fetch all product
foreach (var product in listProduct)
{ //For each product create a html block
dataHTML.Append("<div class='col-lg-3'>");
dataHTML.Append("<div class='panel panel-default'>");
dataHTML.Append(string.Format("<div class='panel-heading'>{0}</div>", product.productLabel));
dataHTML.Append("<div class='panel-body'><center><img src='/Content/images/image.jpg'/></center>");
dataHTML.Append(string.Format("<p>{0}</p></div></div></div>", product.productDescription));
}
return dataHTML.ToString();
}
3) Scenario
- Initial State :

- After clicking on Scan button :

Summary
The main aim of this article is to explain how we can load dynamic data into our web page using Ajax concept.To see another example on this topic, you can follow this link :
I hope that you appreciated my effort. Thank you for viewing my blog post, try to download the source code and do not hesitate to leave your questions and comments.
No comments:
Post a Comment